Java Comments: Methods to Write Documentation
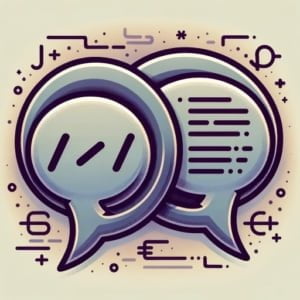
Ever found yourself puzzled over Java comments? You’re not alone. Many developers find themselves in a maze when it comes to effectively using comments in Java. Think of Java comments as your personal code diary – a tool that helps you and others understand your code better.
This guide will walk you through the ins and outs of using comments in Java, from the basics to advanced techniques. We’ll cover everything from creating single-line and multi-line comments, to utilizing Javadoc comments for generating documentation, and even exploring alternative approaches for commenting in Java.
So, let’s dive in and start mastering Java comments!
TL;DR: How Do I Write Comments in Java?
In Java, you can create a single-line comment using
//
and a multi-line comment using/*
and*/
.
Here’s a simple example:
// This is a single-line comment
/* This is a
multi-line comment */
In this example, we’ve used //
to create a single-line comment. Anything written after //
on the same line is considered a comment. For multi-line comments, we’ve used /*
to start the comment and */
to end it. Everything in between is part of the comment.
This is a basic way to use comments in Java, but there’s much more to learn about commenting in Java. Continue reading for more detailed information and advanced usage scenarios.
Table of Contents
- Basic Java Comments: Single-Line and Multi-Line
- Javadoc Comments: Documentation Generation
- Exploring Alternative Commenting Techniques in Java
- Troubleshooting Java Comments: Common Issues and Solutions
- The Importance of Java Comments
- Comments in Large-Scale Software Development
- Exploring Related Concepts
- Wrapping Up: Java Comments
Basic Java Comments: Single-Line and Multi-Line
Comments in Java are essential for improving code readability and understanding the functionality of different code sections. There are two basic types of comments in Java: single-line and multi-line.
Single-Line Comments
A single-line comment in Java starts with //
. The Java compiler ignores everything from //
to the end of the line. Here’s an example:
// This is a single-line comment
System.out.println("Hello, World!");
// Output:
// Hello, World!
In this code block, the System.out.println("Hello, World!");
line is not part of the comment and is executed by the Java compiler. The text This is a single-line comment
is a comment and is ignored by the compiler.
Multi-Line Comments
Multi-line comments start with /*
and end with */
. Everything between these symbols is considered a comment. Here’s an example:
/* This is a
multi-line comment */
System.out.println("Hello, Java!");
// Output:
// Hello, Java!
In this code block, System.out.println("Hello, Java!");
is executed by the compiler, while the text between /*
and */
is a comment and is ignored.
Utilizing comments in your Java code can greatly enhance its readability, making it easier for others (and future you) to understand. However, be careful not to overuse comments or state the obvious, as this can make the code more cluttered and harder to read. The best comments often explain why something is done a certain way, not what is being done.
Javadoc Comments: Documentation Generation
As you advance in your Java journey, you’ll encounter Javadoc comments. These are a special type of multi-line comment used to generate official documentation for your Java classes, methods, and variables.
How to Use Javadoc Comments
Javadoc comments begin with /**
and end with */
. The Java compiler ignores these comments, but the Javadoc tool uses them to generate HTML documentation.
Here’s a simple example:
/**
* This is a Javadoc comment.
* It can span multiple lines and the Javadoc tool will process it.
*/
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
// Output:
// Hello, World!
In this example, the Javadoc comment is associated with the HelloWorld
class. When we run the Javadoc tool on this code, it generates an HTML file with the comment as part of the documentation for the HelloWorld
class.
Understanding Javadoc Comments
Javadoc comments can include HTML tags for formatting and special tags (starting with @) for additional information, such as @param
to describe method parameters, @return
to describe return values, and @author
to name the author.
Using Javadoc comments effectively is a good practice in Java coding, especially for large projects. These comments help maintain a standard documentation format and make it easier for other developers to understand your code.
Exploring Alternative Commenting Techniques in Java
Beyond the basic and Javadoc comments, Java offers other advanced methods for commenting. These include using annotations and third-party documentation tools. Let’s delve into these alternatives to give you a broader perspective on commenting in Java.
Java Annotations
Java annotations are metadata about the code, which can be used by the compiler or runtime environment. They are not comments per se, but they can provide additional information about your code. Here’s an example:
@Override
public String toString() {
return "This is a toString method";
}
// Output:
// This is a toString method
In this example, the @Override
annotation indicates that the toString
method is overriding the method from its superclass. This provides useful information to other developers and the compiler.
Third-Party Documentation Tools
There are also third-party tools available for documenting your Java code. Tools like Doxygen, JavadocDoclet, and Doclet are popular choices. These tools offer more features and customization options than standard Javadoc comments.
For instance, Doxygen supports multiple output formats and can even generate diagrams from your code. Here’s a simple example of a Doxygen comment:
/**
* @file HelloWorld.java
* @author John Doe
* @brief This is a brief description of the HelloWorld class
*
* @details This is a detailed description of the HelloWorld class.
* It can span multiple lines.
*/
public class HelloWorld {
public static void main(String[] args) {
System.out.println("Hello, World!");
}
}
// Output:
// Hello, World!
In this example, we’ve used Doxygen’s @file
, @author
, @brief
, and @details
tags to provide additional information about the HelloWorld
class.
While these alternative approaches to commenting in Java offer more flexibility and features, they may also require additional setup and learning. Therefore, it’s important to choose the right method based on your project requirements and team preferences.
Troubleshooting Java Comments: Common Issues and Solutions
While using comments in Java, you might encounter some common issues. Let’s discuss these problems and their solutions to help you avoid potential pitfalls.
Misplaced Comments
One common issue is misplaced comments. Comments should be placed in a way that makes it clear which code they refer to. Misplaced comments can lead to confusion. Here’s an example of a misplaced comment and its correction:
// Misplaced comment
System.out.println("Hello, World!"); // This is a Hello World program
// Corrected comment
// This is a Hello World program
System.out.println("Hello, World!");
// Output:
// Hello, World!
In the corrected example, it’s clear that the comment refers to the System.out.println("Hello, World!");
line.
Over-Commenting
Over-commenting is another common issue. Too many comments can make code hard to read. Remember, comments should explain why something is done a certain way, not what is being done. Here’s an example of over-commenting and its correction:
// Over-commenting
// This is a Hello World program
// It prints Hello, World! to the console
System.out.println("Hello, World!"); // This line prints Hello, World!
// Corrected comment
// This is a Hello World program
System.out.println("Hello, World!");
// Output:
// Hello, World!
In the corrected example, we’ve removed the redundant comments. The remaining comment provides enough information about the code.
These are just a few examples of common issues when using comments in Java. By being aware of these issues and their solutions, you can use comments more effectively and avoid common pitfalls.
The Importance of Java Comments
Understanding the fundamentals of commenting in Java is crucial for any programmer. Comments are an integral part of coding, enhancing the readability and maintainability of your code.
Enhancing Code Readability
Comments make your code more readable. They provide context and explain the functionality of different sections of your code. This is especially useful when you’re working in a team or when you revisit your old code. Here’s an example:
// Calculate the sum of two numbers
int sum = a + b;
// Output:
// The sum of a and b
In this code block, the comment explains what the line of code does, making it easier to understand.
Improving Code Maintainability
Comments also improve the maintainability of your code. They can explain why a certain approach was used, any limitations, or any pending improvements. This information can be very helpful when you need to update or refactor your code. Here’s an example:
// Using bubble sort because the array size is small
// For larger arrays, consider using more efficient algorithms like quicksort or mergesort
bubbleSort(array);
// Output:
// Sorted array
In this example, the comments provide important information about the choice of the sorting algorithm and potential improvements.
Best Practices for Commenting in Java
While commenting is essential, it’s also important to follow best practices. Comments should be concise and to the point. They should explain why something is done a certain way, not what is being done. Avoid over-commenting or stating the obvious, as this can clutter your code. Also, make sure your comments are up-to-date and reflect the current state of your code.
Comments in Large-Scale Software Development
In large-scale software development, comments become even more vital. They facilitate understanding among team members and help manage complex codebases. Comments are also crucial during code reviews, as they provide context and make the review process more efficient.
Exploring Related Concepts
While mastering Java comments is important, it’s also beneficial to explore related concepts like code documentation and code style. Code documentation involves creating comprehensive and clear documents explaining your codebase, while code style refers to the conventions and guidelines followed when writing code.
Code Documentation
Code documentation is more than just comments in your code. It includes standalone documents explaining the architecture, modules, functions, and more. Good code documentation makes your codebase easier to understand and maintain.
Code Style
Code style involves following certain conventions when writing code. This can include naming conventions, indentation, use of spaces or tabs, and more. A consistent code style makes your code more readable and professional.
Further Resources for Mastering Java Comments
To deepen your understanding of Java, you can Click Here to discover the importance of Java in server-side programming.
For more resources on Java comments and related concepts, here are some helpful guides:
- Common Java Interview Questions – Learn how to approach and answer frequently asked interview questions effectively.
Understanding Java Methods – Learn about method declaration, invocation, parameters, return types, and visibility modifiers
Oracle’s Java Tutorials – A comprehensive guide to Java, including a section on comments.
Google Java Style Guide explains how to style your Java code, including best practices for commenting.
Java Code Conventions – Oracle’s official code conventions for the Java programming language.
Wrapping Up: Java Comments
In this comprehensive guide, we’ve embarked on a journey through the world of Java comments, exploring their importance and the different ways to use them effectively.
We began with the basics, understanding how to create single-line and multi-line comments in Java. We then delved into more advanced territory, learning about Javadoc comments and how they can be used to generate documentation. We also tackled common issues you might encounter when using comments in Java, such as misplaced comments and over-commenting, providing solutions to help you avoid these pitfalls.
We didn’t stop there. We explored alternative methods for commenting in Java, including using annotations and third-party documentation tools, giving you a broader perspective on commenting in Java. Here’s a quick comparison of these methods:
Method | Pros | Cons |
---|---|---|
Single-line and Multi-line Comments | Simple, easy to use | Limited in information they can provide |
Javadoc Comments | Can generate documentation, support HTML and special tags | More complex, may require additional setup |
Annotations | Provide additional metadata about code | Not comments per se, may not be suitable for all use cases |
Third-Party Tools | Offer more features and customization options | Require additional setup and learning |
Whether you’re just starting out with Java or you’re looking to level up your commenting skills, we hope this guide has given you a deeper understanding of Java comments and their importance in coding.
With a firm grasp of Java comments, you’re now equipped to write clearer, more understandable code. Happy coding!